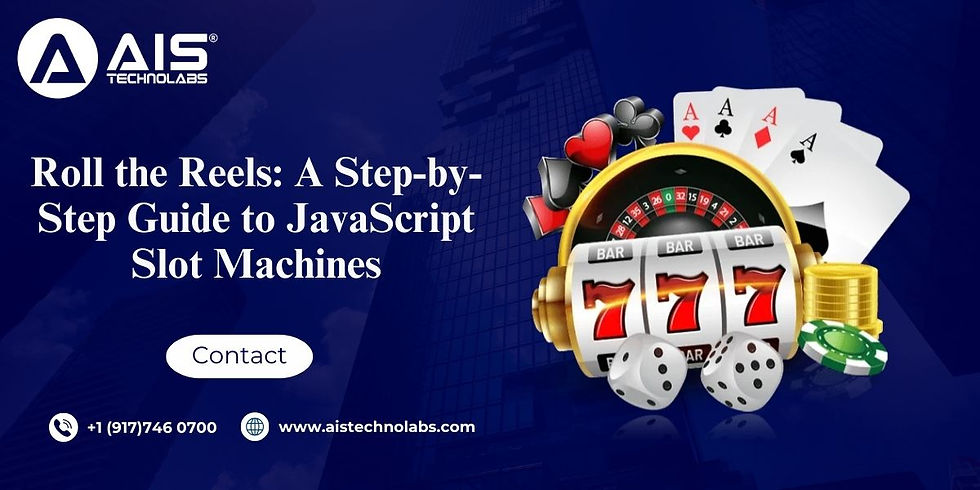
Creating a JavaScript slot machine code is a fun and educational project. It helps you understand core programming concepts while building an interactive game. This guide will walk you through the process of making a slot machine using JavaScript. By the end, you will have a working game that you can customize and expand. Whether you're a beginner or an experienced developer, this guide provides clear and simple instructions to help you build your own slot machine.
Step 1: Plan the Game Structure
Before writing any code, it’s important to plan the structure of your slot machine. Start by deciding how many reels and symbols you want. Common symbols include fruits like cherries, lemons, and oranges, as well as bells and stars. Think about how the game will look and function. Sketch a simple layout to visualize the design.
Consider the following questions:
How many reels will your slot machine have?
What symbols will appear on the reels?
How will the player interact with the game?
What happens when the player wins?
Planning ahead ensures that your development process is smooth and organized.
Step 2: Set Up the HTML
Once you have a clear plan, start by creating the basic HTML structure for your slot machine. Use <div> elements to represent the reels and a button to trigger the spin. Ensure the layout is clean and easy to understand.
Here’s an example of what your HTML might look like:
A container for the slot machine.
Three reels, each displaying a symbol.
A spin button to start the game.
This step focuses on the visual foundation of your game. Keep the HTML simple and well-structured to make it easier to style and add functionality later.
Step 3: Style the Game with CSS
After setting up the HTML, use CSS to style your slot machine. Center the reels and button on the screen. Use colors, borders, and fonts to make the game visually appealing. Keep the design simple and user-friendly.
Consider the following styling elements:
Background color for the game container.
Borders and padding for the reels.
Font size and color for the symbols.
Hover effects for the spin button.
This step ensures your game looks polished and professional. A well-designed interface enhances the player’s experience.
Step 4: Add JavaScript Logic
The next step is to write the JavaScript code to handle the game logic. Use functions to spin the reels and check for winning combinations. Add event listeners to make the game interactive.
Here’s an overview of the JavaScript logic:
Create an array of symbols that will appear on the reels.
Write a function to randomly select a symbol for each reel.
Use set Interval to animate the spinning reels.
Use set Timeout to stop the reels after a few seconds.
Check if the symbols on the reels match to determine a win.
This step brings your slot machine to life. The JavaScript code makes the game functional and interactive.
Step 5: Test and Debug
After writing the code, test your slot machine to ensure it works correctly. Check for any errors or bugs in the code. Make adjustments as needed to improve the game’s performance.
Here are some testing tips:
Test the spin button to ensure it triggers the reels.
Check if the reels stop correctly and display random symbols.
Verify that the winning logic works as expected.
Test the game on different devices and browsers.
This step ensures your game runs smoothly and provides a seamless experience for players.
Step 6: Enhance the Game
Once your slot machine is functional, consider adding extra features to make it more engaging. These additions can improve the player’s experience and make your game stand out.
Here are some ideas for enhancements:
Add sound effects for spinning reels and winning combinations.
Include animations to make the reels spin more realistically.
Add a score counter to track the player’s wins.
Introduce a betting system to make the game more challenging.
Create a leaderboard to encourage competition.
These features make the game more enjoyable and immersive for players.
Step 7: Optimize for Performance
Optimizing your slot machine ensures it runs smoothly and efficiently. This step involves improving the code and design to enhance performance.
Here are some optimization tips:
Minimize the use of heavy animations or large image files.
Use efficient algorithms for random symbol selection.
Test the game on different devices to ensure compatibility.
Compress images and audio files to reduce loading times.
Optimization ensures your game performs well, even on low-end devices.
Step 8: Publish and Share
Once your slot machine is complete, publish it online so others can play. You can host the game on a personal website or share it on coding platforms like Code Pen or GitHub.
Here’s how to publish your game:
Upload the HTML, CSS, and JavaScript files to a web server.
Test the game on the live server to ensure it works correctly.
Share the link with friends, family, or the coding community.
Publishing your game allows others to enjoy your creation and provides an opportunity to receive feedback.
Conclusion
Building a JavaScript slot machine is a great way to practice programming skills. This guide provides a clear and simple approach to creating a functional game. By following these steps, you can create a slot machine that is both fun and interactive. Experiment with different features and designs to make the game your own.
Whether you’re a beginner or an experienced developer, this project offers valuable learning opportunities. Start building your slot machine today and enjoy the process of creating something unique and engaging. For professional assistance in building interactive games, AIS Technolabs. They specialize in creating engaging and high-quality digital solutions. Contact us today to bring your game ideas to life!
FAQs
Q1: Can I use images instead of text for the symbols?
Yes, you can replace text symbols with images. Use image files and update the code to display them on the reels.
Q2: How can I make the game more challenging?
Increase the number of reels or add more symbols. You can also adjust the winning conditions to make the game harder.
Q3: Can I use this for a real casino game?
This guide is for educational purposes. Real casino games require compliance with legal regulations and advanced features.
Q4: How do I add sound effects?
Use JavaScript to play sound files when the reels spin or when the player wins. This adds an immersive element to the game.
Q5: How can I make the game mobile-friendly?
Use responsive design techniques to ensure the game works well on all screen sizes. Test the game on mobile devices and make adjustments as needed.
Comments